Python is an interpreted, interactive, object-oriented, dynamic and strongly typed programming language that is used for a wide range of applications. It incorporates modules, exceptions, dynamic typing, very high-level dynamic data types, and classes. Python combines remarkable power with very clear syntax. It has interfaces to many system calls and libraries, as well as to various window systems, and is extensible in C or C++. It is also usable as an extension language for applications that need a programmable interface. Finally, Python is portable: it runs on many Unix variants, on the Mac, and on Windows 2000 and later.
The language comes with a large standard library that covers areas such as string processing (regular expressions, Unicode, calculating differences between files), Internet protocols (HTTP, FTP, SMTP, XML-RPC, POP, IMAP, CGI programming), software engineering (unit testing, logging, profiling, parsing Python code), and operating system interfaces (system calls, filesystems, TCP/IP sockets). Look at the table of contents for The Python Standard Library to get an idea of what’s available. A wide variety of third-party extensions are also available. Consult the Python Package Index to find packages of interest to you.
Python allows programmers to express concepts in fewer lines of code than would be possible in many other languages, such as C, and the language has constructs intended to be used to create clear programs in a variety of domains.
Example:
Python program
print("hello stackoverflow")
versus
C program
#include <stdio.h>
int main(void) {
printf("hello stackoverflow");
return 0;
}
Python was originally created by Guido van Rossum and first released in 1991. Guido Van Rossum chose Python as a working title for the project, being in a slightly irreverent mood (and a big fan of Monty Python's Flying Circus).
Two similar, though incompatible, versions of Python are in widespread use, Python 2 (16 October 2000) and Python 3 (3 December 2008). Please consider mentioning the version and implementation that you are using, when asking a question about Python (see Tagging Recommendation section below).
Python supports multiple programming paradigms, including object-oriented, imperative, and functional programming styles. It features a fully dynamic type system and automatic memory management, similar to that of Scheme, Ruby, Perl, and Tcl.
Like other dynamic languages, Python is often used as a scripting language but is also used in a wide range of non-scripting contexts. Using third-party tools, Python code can be packaged into standalone executable programs. Python interpreters are available for many operating systems.
CPython, the reference implementation of Python, is free and open-source software. It has a community-based development model, as do nearly all of its alternative implementations. There are a wide variety of implementations more suited for specific environments or tasks (see Python implementations on the Python wiki).
The philosophy of Python is succinctly formulated in The Zen of Python, written by Tim Peters, which one can read by issuing this command, in the interactive python interpreter:
>>> import this
Unlike many other languages, Python uses an indentation-based syntax (in which tabs and spaces are noninterchangeable). This may take some getting used to for programmers who are familiar with using braces.
>>> from __future__ import braces
File "<stdin>", line 1
SyntaxError: not a chance
>>>
To help with the transition, using a properly configured text-editor or IDE is recommended. Python comes with a basic IDE called IDLE (python-idle), to get you started. Other popular examples are the charityware Vim, the free GNU Emacs, Eclipse+PyDev, or PyCharm. Take a look at this IDE comparison list for many other alternatives.
There is also a style guide for Python, named PEP 8, which aims to make Python code more readable and consistent. This guide is (should be) followed all across the Python development community.
Tagging Recommendation:
Use the python tag, for all Python-related questions. If you believe your question includes issues specific to individual versions, use python-3.x or python-2.7, in addition to the main python tag. If you believe your question maybe even more specific, you can include a version-specific tag such as python-3.5 or python-3.6, etc.
Also, consider including the tag for the specific implementation (jython, pypy, etc.), if you are using one other than cpython — the use of cpython is assumed unless explicitly stated otherwise.
References:
- Official documentation for the current stable versions: 2.7.x and 3.8.x.
- Release notes for the current stable versions: 2.7.16 and 3.8.0.
- Python (programming language) (Wikipedia)
- Python for Programmers
- Python - Quick Guide
- Getting started with Python
- Porting Python 2 Code to Python 3
- 2to3 - Automated Python 2 to 3 code translation
- The non-profit Python Software Foundation manages CPython.
- PSF License Agreement for Python 2.7.x and 3.8.x
- Awesome-Python
- Full Stack Python
- Python Read the docs
Popular general use Python libraries:
- Requests requestsA simple Python library for making HTTP requests. Requests are marketed as being "For Humans". The library is meant to simplify and universalize Python's many methods for making HTTP requests in a way that is readable and easy to use. Functionality such as keep-alive and connection pooling are automatically handled to provide ultimate simplicity.
- Pillow python-imaging-libraryPillow is described as being a "friendly fork" of the Python PIL module, an unmaintained but useful imaging library. The library uses C APIs to provide an easy Python interface to modify and manipulate image files in many different ways.
- Scrapy scrapyScrapy is a fast high-level web crawling and web scraping framework used to crawl websites and extract structured data from their pages. It can be used for a wide range of purposes, from data mining to monitoring and automated testing.
- Beautiful Soup beautifulsoupBeautiful Soup is a Python package for parsing HTML and XML documents. It creates a parse tree for parsed pages that can be used to extract data from HTML, which is useful for web scraping. It is available for Python 2.7 and Python 3.
- nltk nltkThe Natural Language Toolkit, or NLTK, is a platform for building Python applications to work with human language data and the processing of sentences. It provides easy-to-use interfaces to over 50 corpora and lexical resources such as WordNet, along with a suite of text processing libraries for classification, tokenization, stemming, tagging, parsing, and semantic reasoning, and providing wrappers for industrial-strength NLP libraries.
Popular web frameworks based on Python:
If your question has anything to do with any of these frameworks, please ensure you include the appropriate tag.
- Django djangoThe Web framework for perfectionists (with deadlines). Django makes it easier to build better Web apps more quickly and with less code. Django is a high-level Python Web framework that encourages rapid development and clean, pragmatic design. It lets you build high-performance, elegant Web applications quickly. Django focuses on automating as much as possible and adhering to the DRY (Don't Repeat Yourself) principle.
- Flask flaskFlask is a lightweight micro-framework and third party library for Python based on Werkzeug, Jinja 2 and good intentions. It provides monolithic structure and does not enforce dependencies which allows for finer control and higher freedom for development.
- Quart quartThe quart is an evolution of the Flask API to work with Asyncio and to provide a number of features not present or possible in Flask.
- Tornado tornadoTornado is a Python web framework and asynchronous networking library. By using the non-blocking network I/O, Tornado can scale to tens of thousands of open connections, making it ideal for long polling, WebSockets, and other applications that require a long-lived connection to each user.
- CherryPy cherrypyCherryPy is a pythonic, object-oriented web framework that enables developers to build web applications, in much the same way they would build any other object-oriented Python program. This results in a smaller amount of source code which is developed in less time. CherryPy has been in use for over 17 years and it is being used in production by many sites, from the simplest to the most demanding.
- Pyramid pyramidA lightweight web framework emphasizing flexibility and rapid development. It combines the very best ideas from the worlds of Ruby, Python and Perl, providing a structured but extremely flexible Python web framework. It's also one of the first projects to leverage the emerging WSGI standard, which allows extensive re-use and flexibility, but only if you need it.
- TurboGears turbogearsTurboGears is a scalable web framework, which can go from a minimal mode setup to a full-stack web application. It was created in 2005 by Kevin Dangoor, and the current development of TurboGears2 (turbogears2) is being led by Mark Ramm. The current stable release of TurboGears is TurboGears 2.3.12, released April 6th 2018
- web.py web.pyweb.py is a web framework for Python that is as simple as it is powerful. web.py is in the public domain: you can use it for whatever purpose with absolutely no restrictions. web.py lets you write web apps in Python.
- Grok grokBuilt on the existing Zope 3 libraries but aims to provide an easier learning curve and a more agile development experience. Grok does this by placing an emphasis on convention over configuration and DRY (Don't Repeat Yourself).
- Bottle bottleThe bottle is a fast, simple and lightweight WSGI micro web-framework for Python. It is distributed as a single file module and has no dependencies other than the Python Standard Library.
- web2py web2pyweb2py is a free open source full-stack framework for rapid development of fast, scalable, secure and portable database-driven web-based applications.
- Falcon falconframeworkFalcon is a minimal Python web framework for building microservices, app backends, and higher-level frameworks and encourages the REST architectural style. It has both community and commercial versions.
- Twisted twistedTwisted is an open-source event-driven networking engine. It is useful for implementing both clients and servers and scales up to large websites and down to embedded devices. Twisted makes it easy to implement custom network applications.
Popular Python GUI Frameworks based on Python
- Kivy kivyKivy is an OpenGL ES 2 accelerated framework for the creation of new user interfaces. It supports multiple platforms namely Windows, MacOSX, Linux, Android iOS, and Raspberry Pi. It is open source and comes with over 20 widgets in its toolkit.
- PyQT pyqtPyQT is one of the favoured cross-platform Python bindings implementing the Qt library for the Qt (owned by Nokia) application development framework. Currently, PyQT is available for Unix/Linux, Windows, Mac OSX, and Sharp Zaurus. It combines the best of Python and Qt and it up to the programmer to decide whether to create a program by coding or using Qt Designer to create visual dialogs.It is available in both commercial as well as GPL license. Although some features may not be available in the free version, if your application is open source, then you can use it under the free license.
- Tkinter tkinterTkinter is commonly bundled with Python, using Tk and is Python’s standard GUI framework. It is popular for its simplicity and graphical user interface. It is open-source and available under the Python License. One of the advantages of choosing Tkinter is that since it comes by default, there is an abundance of resources, both codes and reference books. Also with the community being old and active, there are many users who can help you out in case of questions.
- PyGUI pyguiPyGUI is a graphical application cross-platform framework for Unix, Macintosh, and Windows. Compared to some other GUI frameworks, PyGUI is by far the simplest and lightweight of them all, as the API is purely in sync with Python. PyGUI inserts very little code between the GUI platform and your Python application, hence the display of the application usually displays the natural GUI of the platform.
Popular Mathematical/Scientific computing libraries in Python
- NumPy numpyNumPy is the fundamental package for scientific computing with Python. It contains among other things:a powerful N-dimensional array objectsophisticated (broadcasting) functionstools for integrating C/C++ and Fortran codeuseful linear algebra, Fourier transform, and random number capabilitiesThese features also make it possible to use NumPy in general-purpose database applications.
- SciPy scipySciPy is an open-source library for the Python programming language, consisting of mathematical algorithms and functions often used in science and engineering. SciPy includes algorithms and tools for tasks such as optimization, clustering, discrete Fourier transforms, linear algebra, signal processing, and multi-dimensional image processing. SciPy is closely related to NumPy and depends on many NumPy functions, including a multidimensional array that is used as the basic data structure in SciPy.
- matplotlib matplotlibmatplotlib is a plotting library for the Python programming language and its NumPy numerical mathematics extension. It provides an object-oriented API for embedding plots into applications, using general-purpose GUI toolkits like wxPython, Qt, or GTK. There is also a procedural "pylab" interface, based on a state machine (like OpenGL), designed to closely resemble that of MATLAB.
- pandas pandasPandas is an open-source BSD-licensed library providing high-performance, easy to use data structures and data analysis tools for the Python programming language. Pandas integrates many other libraries' features, namely NumPy's matrix operations, and Matplotlib's plotting capabilities. 10 Minutes to Pandas is a good tutorial for first exposure to Pandas.
- theano theanoTheano is a Python-C-based widely-used library suitable for highly computational mathematical tasks due to the optimizations it does on the interface Python code making it highly optimized using its C-based routines. It is a very popular library for machine-learning researchers as well. It features a highly optimized automatic differentiation, easing the implementations of highly complicated functions and computing their gradients without any errors.
- Blender blenderBlender is a free and open-source 3D animation suite. It supports the entirety of the 3D pipeline—modeling, rigging, animation, simulation, rendering, compositing and motion tracking, even video editing and game creation.
- scikit-learn scikit-learnscikit-learn is a free and open-source machine learning library written in Python. It supports training and testing many different kinds of machine learning models, along with some basic data processing techniques.
- TensorFlow tensorflowTensorFlow is an open-source software library, developed by the Google Brain team. It is a symbolic math library, used mostly for machine learning applications, such as neural networks.
Popular C extension solutions:
With C extension, you can make your python code faster. If your question has anything to do with any of the next solutions, please ensure you include the appropriate tag.
- ctypes ctypes
ctypes
is a Python package that wraps C .dll/.so libraries in pure Python. - swig swigSWIG is an interface compiler that connects programs written in C and C++ with scripting languages such as Python.
- cython cythonCython is an optimising static compiler for both the Python programming language and the extended Cython programming language (based on Pyrex). It makes writing C extensions for Python as easy as Python itself.
Community
Chat Rooms
- Chat at the dedicated IRC channel #python on Freenode for all things Python. Look at Python IRC listing for a specific alternative channel, if interested.
- Chat about Python with other Stack Overflow users in the Python chat room.
Other Sites
- The Python Package Index - The Python Package Index (PyPI) is a repository of software for the Python programming language.
- Tutor mailing list
- python-help mailing list
- PyCon
- Python Weekly
- Pycoder's Weekly
- Python Google Group
- Python Subreddit
- learnpython Subreddit
- Hacker Rank - Solving problems with Python
- Real Python - Python Tutorials & Newsletter
- Python Course - Python tutorials
Free Python Programming Books
- Object Oriented Design in Python
- Wikibooks' Non-Programmers Tutorial for Python 2.6
- Wikibooks' Non-Programmers Tutorial for Python 3
- The Official Python Tutorial
- Building Skills in Python Version 2.6 (Steven F. Lott)
- A Byte of Python (Swaroop C H.)
- Problem-Solving with Algorithms and Data Structures using python (Brad Miller and David Ranum)
- Dive into Python 3
- Invent Your Own Computer Games With Python (Al Sweigart)
- Making Games with Python & Pygame (Albert Sweigart)
- Natural Language Processing with Python (Steven Bird, Ewan Klein, and Edward Loper)
- Python Bibliotheca
- Python for Fun (Chris Meyers)
- Snake Wrangling For Kids (Jason R. Briggs)
- Think Python (PDF file) (Allen Downey)
- Think Python 3 (Allen Downey)
- Porting to Python 3 (Lennart Regebro)
- Automate the Boring Stuff with Python (Al Sweigart)
- Python® Notes for Professionals book(GoalKicker)
- Python Practice Book(Anand Chitipothu)
Interactive Python Learning
- Codecademy - Learn the fundamentals of Python and dynamic programming
- CodeSkulptor - Interactive online IDE for Python 2 programming
- CodeSkulptor 3 - Interactive online IDE for Python 3 programming
- Coursera - Online course for introduction to interactive Python programming
- CheckiO - A game world you can explore, using your Python programming skills
- Repl.it - Online interpreter for Python 2 and 3 that simplifies saving and sharing code
- PyCharm Edu - A desktop application that offers interactive Python learning
- Interactive Python - Includes a modified, interactive version of How to Think Like a Computer Scientist
- Python Tutor - Visualization and/or live coding in Python
- Computer Science Circles - Learn basic Python 3 in a semi-interactive fashion.
Python Online Courses
- Interactive Programming With Python - Introduction to interactive programming with python.
- Programming for Everybody - Introduction to programming using Python.
- Introduction to Computer Science and Programming Using Python - A new and updated introduction to computer science as a tool to solve real-world analytical problems, using Python 3.
- Intro to Computer Science - Explore computer science basics, as you build your own search engine and social network while learning Python.
Python Video Tutorials
Python for Scientists
Python Online IDE
- ideone - An online IDE, with other popular language support.
- repl - Instant programming environment for your favorite language
- python shell - Online console from PythonAnywhere
- pythonfiddle - Python Cloud IDE
- pyfiddle - Python 2.7/3.6 online console
Code Quality
- Codacy - Automated Code Review to ship better code, faster.
- Codecov - Code coverage dashboard.
- CodeFactor - Automated Code Review for Git.
- Landscape - Hosted continuous Python code metrics.
Official Logo:
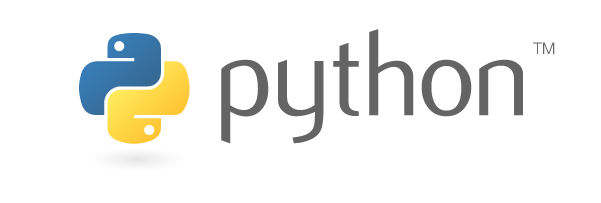